Heater with temperature controller for solar wax melters
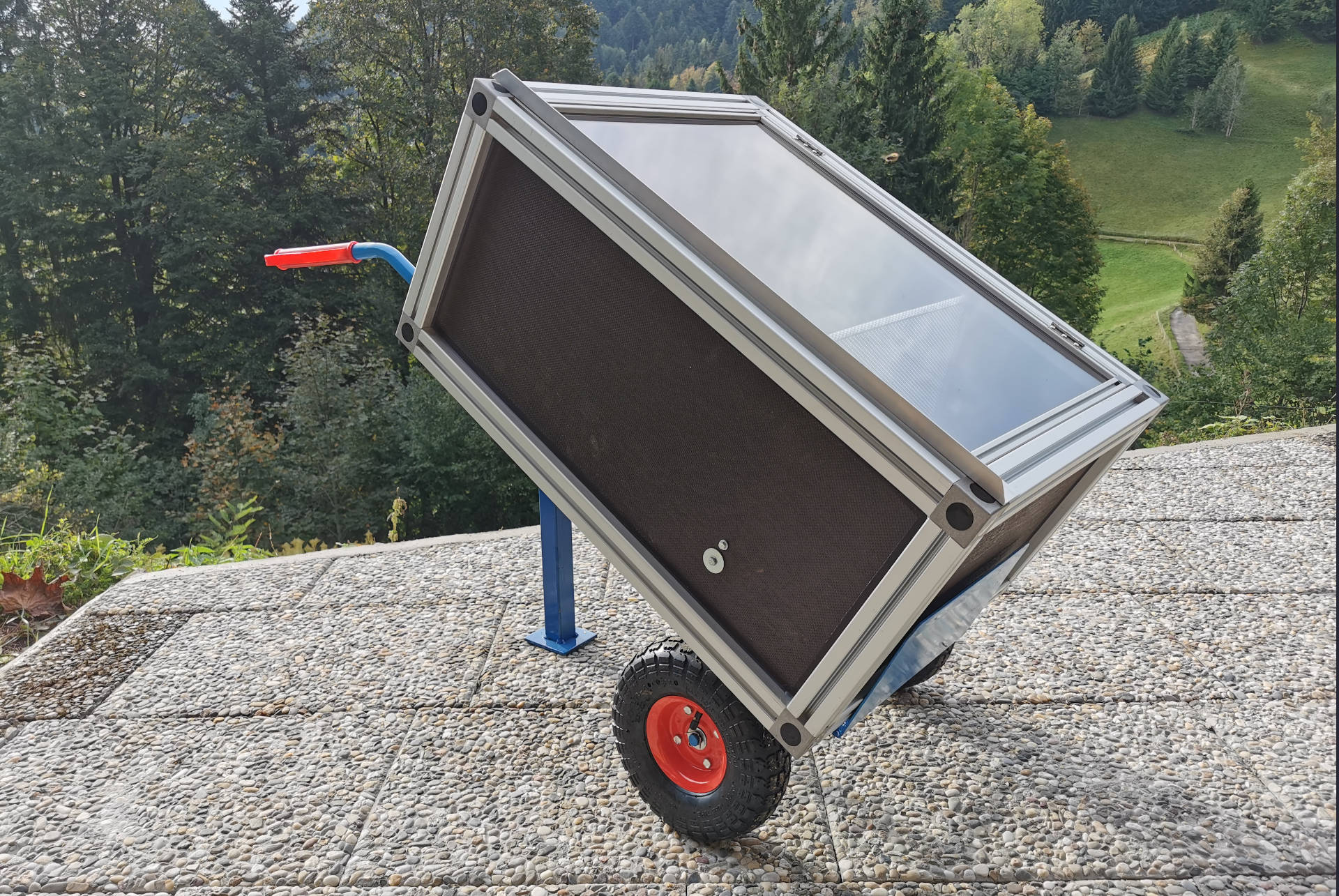
Materialliste
Description
In summer, a solar wax melter is the best way for me to melt old honeycombs immediately. Since bees are often foraging during this time, it's difficult to use a steam wax melter or steam wax extractor. Furthermore, these devices are often only worthwhile for large quantities of old honeycombs. The effort required to clean the devices is too great to melt just a few combs, and storing the combs for a longer period until a larger quantity is collected is impossible due to wax moths.
To install the heater, I used my solar wax melter, which unfortunately only generated sufficient heat on extremely hot summer days. The solar wax melter has space for 10 combs and, thanks to its mounting on a handcart, is mobile despite its size. The only disadvantage of this model is that the outside temperature must be very high for the old combs to melt properly and for the beeswax to flow completely into the tub.
So I came up with the idea that an additional heater could solve this problem, and I quickly considered how to implement simple temperature regulation. Since I already had a lot of experience with the Particle microcontroller boards from the beehive scales, I immediately set up a test setup and wrote the appropriate program.
The heating element requires 220V, which is why I also used the FeatherWing Power Relay from Adafruit and the Particle FeatherWing Tripler for easy wiring. The DHT22 has served me well as a temperature sensor many times, and there's also a suitable program library for it.
The principle of the circuit and the program are incredibly simple. The temperature sensor measures the temperature – if it's below 60°C, the heating element is switched on via the power relay. As soon as the temperature rises above this value, the heating element is switched off again. Since the temperature control doesn't need to be very precise, it's perfectly sufficient to measure the temperature every minute. To show the temperature and humidity levels in the solar wax melter, these values are transmitted to the Particle Console.
Fritzing - circuit diagram
Fritzing - plug-in board:
Installation and programming
To regulate the temperature of the solar wax melter, I use a Wi-Fi microcontroller board from Particle with the FeatherWing design. It doesn't really matter whether I use a Particle Argon or a Photon 2. In principle, a Boron with a 2G/3G network connection could also be used. To use one of these boards, you must first complete the setup process. This involves loading the latest firmware version onto the board and establishing a network connection. You then need to assign the board to a product or create a new product. At the end of the setup, the device appears in the Particle console. Here you can check the device's network status and the state of variables, which must first be set in the program code.
There are two ways to write, compile, and transfer the program code to the Particle device. We can either create the program directly in Particle's Web IDE or install the Particle Workbench in Visual Studio Code. Using the Web IDE is certainly the easiest and fastest way to create a program and transfer it to a Particle device. I would therefore recommend using the Web IDE for this simple program example.
The program code can be divided into three sections. The first section involves incorporating program libraries and defining variables. This is followed by the setup code. This is only executed once and, in this case, involves registering variables that can be accessed in the Particle console in the future.
The third section of the program consists of a loop function that is executed repeatedly. Here, a Wi-Fi connection is first established, then the device is connected to the cloud. The temperature and humidity of the DHT22 sensor are then determined and transmitted to the Particle Cloud. The last section is a simple if statement that turns the heating element on when the temperature falls below 60°C and turns it off when the value rises above this value.
I think the program still has a lot of room for improvement, but it should be sufficient to demonstrate the basic principle of temperature control.
/*
* Project HeatController
* Author: Dieter Metzler
* Date: 03.05.2024
* For comprehensive documentation and examples, please visit:
* https://docs.particle.io/firmware/best-practices/firmware-template/
*/
// Include Particle Device OS APIs
#include "Particle.h"
#include "PietteTech_DHT.h"
#define DHTTYPE DHT22 // Sensor type DHT11/21/22/AM2301/AM2302
#define DHTPIN A0 // Digital pin for communications
// Let Device OS manage the connection to the Particle Cloud
SYSTEM_MODE(SEMI_AUTOMATIC);
//********************************************************************
// Automatically mirror the onboard RGB LED to an external RGB LED
// No additional code needed in setup() or loop()
//Since 0.6.1 Allows a set of PWM pins to mirror the functionality of the on-board RGB LED.
STARTUP(RGB.mirrorTo(D4, D5, D6));
// Show system, cloud connectivity, and application logs over USB
// View logs with CLI using 'particle serial monitor --follow'
SerialLogHandler logHandler;
// We define MY_LED to be the LED that we want to blink.
//
// In this tutorial, we're using the blue D7 LED (next to D7 on the Photon
// and Electron, and next to the USB connector on the Argon and Boron).
const pin_t Heater = D7;
// The following line is optional, but recommended in most firmware.
// allows your code to run before the cloud is connected. In this case,
// it will begin blinking almost immediately instead of waiting until
// breathing cyan,
SYSTEM_THREAD(ENABLED);
// Lib instantiate
PietteTech_DHT DHT(DHTPIN, DHTTYPE);
double tempC = 0;
double hum = 0;
double vol = 0;
float temperature;
float humidity;
float voltage;
// The setup() method is called once when the device boots.
void setup() {
Serial.begin(9600);
Particle.variable("temp", tempC);
Particle.variable("hum", hum);
Particle.variable("vol", vol);
DHT.begin();
// In order to set a pin, you must tell Device OS that the pin is
// an OUTPUT pin. This is often done from setup() since you only need
// to do it once.
pinMode(Heater, OUTPUT);
}
// The loop() method is called frequently.
void loop() {
//WiFi.off(); //Turning off the WiFi module will force it to go through a full re-connect to the WiFi network the next time it is turned on.
delay(500);
WiFi.on(); //Turns on the WiFi module. Useful when you've turned it off, and you changed your mind.
Serial.println("WiFi on...");
delay(500);
WiFi.connect(); // This command turns on the WiFi Modem and tells it to connect to the WiFi network.
Particle.connect(); //Connects the device to the Cloud. This will automatically activate the cellular connection and attempt to connect to the Particle cloud if the device is not already connected to the cloud.
Serial.print(": Retrieving information from sensor: ");
Serial.print("Read sensor: ");
int result = DHT.acquireAndWait(1000); // wait up to 1 sec (default indefinitely)
switch (result) {
case DHTLIB_OK:
Serial.println("OK");
break;
case DHTLIB_ERROR_CHECKSUM:
Serial.println("Error\n\r\tChecksum error");
break;
case DHTLIB_ERROR_ISR_TIMEOUT:
Serial.println("Error\n\r\tISR time out error");
break;
case DHTLIB_ERROR_RESPONSE_TIMEOUT:
Serial.println("Error\n\r\tResponse time out error");
break;
case DHTLIB_ERROR_DATA_TIMEOUT:
Serial.println("Error\n\r\tData time out error");
break;
case DHTLIB_ERROR_ACQUIRING:
Serial.println("Error\n\r\tAcquiring");
break;
case DHTLIB_ERROR_DELTA:
Serial.println("Error\n\r\tDelta time to small");
break;
case DHTLIB_ERROR_NOTSTARTED:
Serial.println("Error\n\r\tNot started");
break;
default:
Serial.println("Unknown error");
break;
}
Serial.print("Humidity (%): ");
Serial.println(DHT.getHumidity(), 2);
humidity = DHT.getHumidity();
hum = humidity;
Serial.println("Humidity: " + String(humidity));
Serial.print("Temperature (oC): ");
Serial.println(DHT.getCelsius(), 2);
temperature = DHT.getCelsius();
tempC = temperature;
Serial.println("Temperature: " + String(temperature));
voltage = analogRead(BATT) * 0.0011224;
vol = voltage;
Serial.println("Voltage: " + String(voltage));
if (temperature <= 60) {
// Turn on the LED
digitalWrite(Heater, HIGH);
} else {
// Turn it off
digitalWrite(Heater, LOW);
}
delay(60000);
}